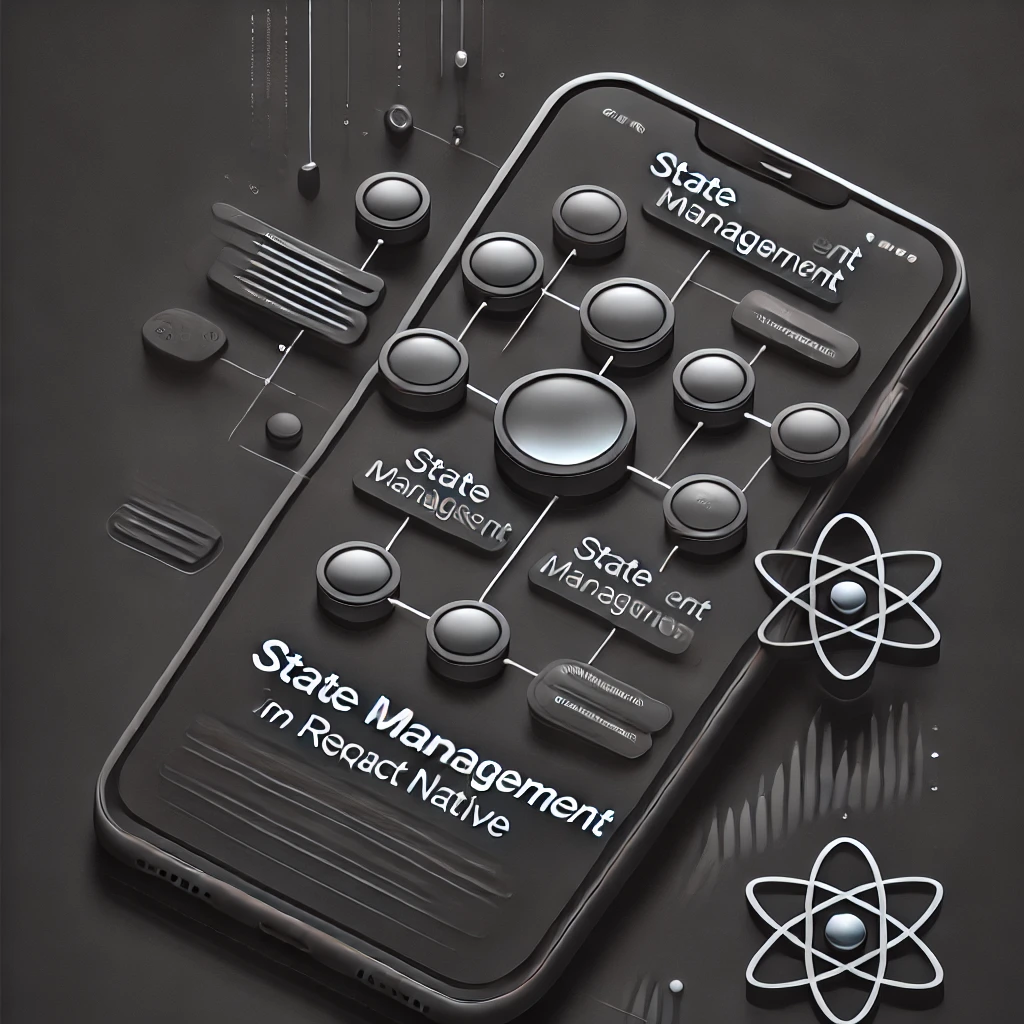
State Management in React Native
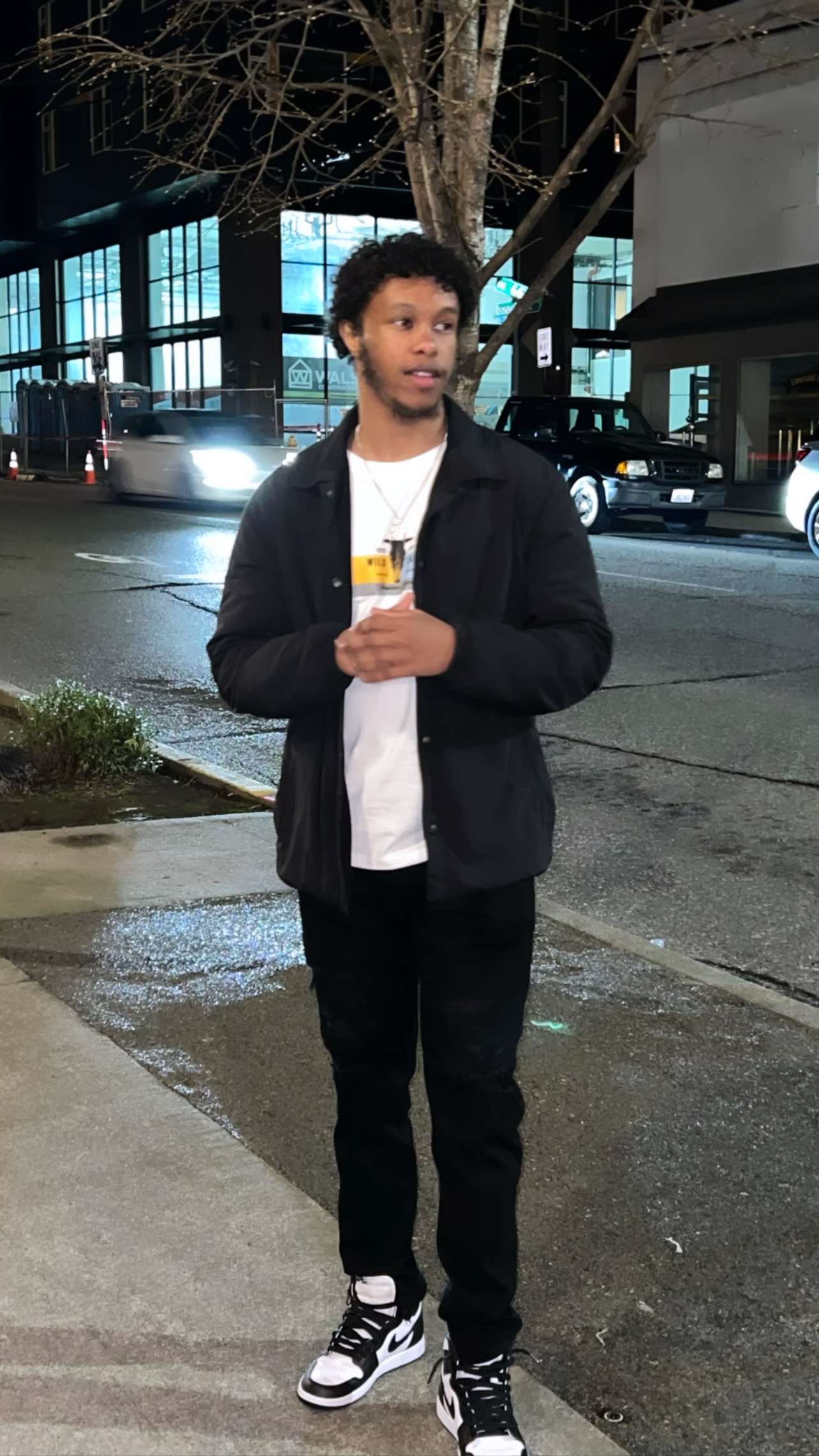
Najib Abdi, November 10, 2024
Introduction
State management is a critical aspect of building scalable and maintainable React Native applications. As mobile applications grow in complexity, sophisticated state management becomes not just beneficial, but crucial. This blog delves deep into advanced techniques for managing state in React Native, with a focus on scalability. We'll focus on three primary methods: Optimized Local State, Context API with Performance Considerations, and Redux with Advanced Patterns.
1. Optimized Local State Management
While local state management might seem basic at first, there are advanced techniques to optimize performance and enhance code readability in complex components.
Using useReducer for Complex State Logic
- When dealing with intricate state logic, 'useReducer' offers a more predictable solution than multiple 'useState' calls.
import React, { useReducer, useCallback } from "react";
import { View, Text, TouchableOpacity, StyleSheet } from "react-native";
const initialState = { count: 0, lastAction: null, history: [] };
const reducer = (state, action) => {
switch (action.type) {
case "increment":
return {
...state,
count: state.count + 1,
lastAction: "increment",
history: [
...state.history,
{ action: "increment", count: state.count + 1 },
],
};
case "decrement":
return {
...state,
count: state.count - 1,
lastAction: "decrement",
history: [
...state.history,
{ action: "decrement", count: state.count - 1 },
],
};
case "reset":
return { ...initialState };
case "undo":
const newHistory = state.history.slice(0, -1);
const lastHistoryItem = newHistory[newHistory.length - 1];
return {
...state,
count: lastHistoryItem ? lastHistoryItem.count : 0,
lastAction: "undo",
history: newHistory,
};
default:
throw new Error();
}
};
const CounterApp = () => {
const [state, dispatch] = useReducer(reducer, initialState);
const handleAction = useCallback((actionType) => {
dispatch({ type: actionType });
}, []);
return (
<View style={styles.container}>
<Text style={styles.counterText}>Count: {state.count}</Text>
<Text style={styles.actionText}>
Last Action: {state.lastAction || "None"}
</Text>
<View style={styles.buttonContainer}>
<TouchableOpacity
style={styles.button}
onPress={()=> handleAction("decrement")}
>
<Text style={styles.buttonText}>-</Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.button}
onPress={()=> handleAction("increment")}
>
<Text style={styles.buttonText}>+</Text>
</TouchableOpacity>
</View>
<TouchableOpacity
style={[styles.button, styles.resetButton]}
onPress={()=> handleAction("reset")}
>
<Text style={styles.buttonText}>Reset</Text>
</TouchableOpacity>
<TouchableOpacity
style={[styles.button, styles.undoButton]}
onPress={()=> handleAction("undo")}
disabled={state.history.length= 0}
>
<Text style={styles.buttonText}>Undo</Text>
</TouchableOpacity>
<Text style={styles.historyText}>
History: {state.history.length} actions
</Text>
</View>
);
};
const styles = StyleSheet.create({
// ... (your own styles)
});
Explanation:
- useReducer Hook: This hook provides a more structured way to manage complex state logic. It's particularly useful when the next state depends on the previous one, or when you have multiple sub-values in your state.
- Reducer Function: The reducer encapsulates all state transition logic, making it easier to understand and test state changes. We've expanded it to include an undo feature and action history.
- Dispatch Actions: Instead of directly setting state, we dispatch actions, which makes state updates more predictable and easier to debug.
- useCallback: We've wrapped our action dispatcher in a 'useCallback' hook to optimize performance by preventing unnecessary re-renders of child components.
When to Use Optimized Local State:
- When component state logic becomes complex with multiple related state variables.
- When you need to optimize performance in larger components with frequent state updates.
- When you want to improve the predictability and testability of your state logic.
- For managing form state, especially in complex forms with multiple fields and validations.
2. Context API with Performance Considerations
For medium-sized applications, the 'Context API' provides a powerful way to manage global state without the complexity of Redux.
Context Splitting for Performance
- Split your context based on how often the data changes to prevent unnecessary re-renders.
import React, { createContext, useContext, useState, useMemo } from "react";
const UserContext = createContext();
const ThemeContext = createContext();
const SettingsContext = createContext();
export const AppProvider = ({ children }) => {
const [user, setUser] = useState(null);
const [theme, setTheme] = useState("light");
const [settings, setSettings] = useState({
notifications: true,
language: "en",
});
const userValue = useMemo(() => ({ user, setUser }), [user]);
const themeValue = useMemo(() => ({ theme, setTheme }), [theme]);
const settingsValue = useMemo(() => ({ settings, setSettings }), [settings]);
return (
<UserContext.Provider value={userValue}>
<ThemeContext.Provider value={themeValue}>
<SettingsContext.Provider value={settingsValue}>
{children}
</SettingsContext.Provider>
</ThemeContext.Provider>
</UserContext.Provider>
);
};
export const useUser = () => useContext(UserContext);
export const useTheme = () => useContext(ThemeContext);
export const useSettings = () => useContext(SettingsContext);
Context with Reducer for Complex State
- Combine the Context API with 'useReducer' for more sophisticated state management scenarios.
import React, { createContext, useContext, useReducer, useMemo } from "react";
const initialState = {
user: null,
isLoading: false,
error: null,
authToken: null,
};
const authReducer = (state, action) => {
switch (action.type) {
case "LOGIN_START":
return { ...state, isLoading: true, error: null };
case "LOGIN_SUCCESS":
return {
...state,
isLoading: false,
user: action.payload.user,
authToken: action.payload.token,
};
case "LOGIN_FAILURE":
return {
...state,
isLoading: false,
error: action.payload,
user: null,
authToken: null,
};
case "LOGOUT":
return initialState;
case "UPDATE_USER":
return { ...state, user: { ...state.user, ...action.payload } };
default:
return state;
}
};
const AuthContext = createContext();
export const AuthProvider = ({ children }) => {
const [state, dispatch] = useReducer(authReducer, initialState);
const login = async (credentials) => {
dispatch({ type: "LOGIN_START" });
try {
// Simulating API call
const response = await fetch("https://api.example.com/login", {
method: "POST",
body: JSON.stringify(credentials),
});
const data = await response.json();
dispatch({ type: "LOGIN_SUCCESS", payload: data });
} catch (error) {
dispatch({ type: "LOGIN_FAILURE", payload: error.message });
}
};
const logout = () => dispatch({ type: "LOGOUT" });
const updateUser = (updates) =>
dispatch({ type: "UPDATE_USER", payload: updates });
const value = useMemo(
() => ({ state, dispatch, login, logout, updateUser }),
[state]
);
return <AuthContext.Provider value={value}>{children}</AuthContext.Provider>;
};
export const useAuth = () => useContext(AuthContext);
Explanation:
- Context Splitting: By separating frequently changing state (like user data) from less frequently changing state (like theme), we prevent unnecessary re-renders of components that only depend on one type of state.
- Context with Reducer: This pattern combines the simplicity of the 'Context API' with the predictability of a reducer, making it easier to manage complex state flows like authentication.
- useMemo for Context Value: We use 'useMemo' to memoize the context value, ensuring that it only changes when the state actually changes, further optimizing performance.
When to Use Context API:
- When you need to share state across multiple components at different levels of the component tree.
- When you want to avoid prop drilling but don't need the full complexity of Redux.
- For managing global UI state like themes or user preferences.
- When you need a lighter-weight solution for global state management in medium-sized applications.
3. Redux for Large-Scale Applications
For complex, large-scale applications, Redux provides a robust solution for state management.
Middleware for Side Effects
- Use middleware like 'Redux Thunk' for handling asynchronous actions.
import { createStore, applyMiddleware } from "redux";
import thunk from "redux-thunk";
import rootReducer from "./reducers";
const store = createStore(rootReducer, applyMiddleware(thunk));
// Async action creator
export const fetchUser = (userId) => async (dispatch) => {
dispatch({ type: "FETCH_USER_START" });
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
const data = await response.json();
dispatch({ type: "FETCH_USER_SUCCESS", payload: data });
} catch (error) {
dispatch({ type: "FETCH_USER_FAILURE", payload: error.message });
}
};
Selectors for Derived State
- Use 'Reselect' for efficient computation of derived data.
import { createSelector } from "reselect";
const getUsers = (state) => state.users;
const getActiveFilter = (state) => state.activeFilter;
export const getActiveUsers = createSelector(
[getUsers, getActiveFilter],
(users, activeFilter) => users.filter((user) => user.status === activeFilter)
);
// Memoized selector with multiple inputs
export const getUsersByRole = createSelector(
[getUsers, (state, role) => role],
(users, role) => users.filter((user) => user.role === role)
);
Redux Toolkit for Simplified Redux Logic
- Leverage 'Redux Toolkit' to reduce boilerplate and streamline development.
import {
configureStore,
createSlice,
createAsyncThunk,
} from "@reduxjs/toolkit";
export const fetchTodos = createAsyncThunk("todos/fetchTodos", async () => {
const response = await fetch("https://jsonplaceholder.typicode.com/todos");
return response.json();
});
const todosSlice = createSlice({
name: "todos",
initialState: { entities: [], loading: "idle" },
reducers: {
todoAdded(state, action) {
state.entities.push(action.payload);
},
},
extraReducers: (builder) => {
builder
.addCase(fetchTodos.pending, (state) => {
state.loading = "loading";
})
.addCase(fetchTodos.fulfilled, (state, action) => {
state.loading = "idle";
state.entities = action.payload;
});
},
});
const store = configureStore({
reducer: {
todos: todosSlice.reducer,
},
});
Summary
This blog post explores three key strategies for managing state in scalable React Native applications:
-
Optimized Local State: Best for smaller components or apps with simple state logic. Uses 'useReducer' for complex state and 'useCallback' for performance.
-
Context API: Suitable for medium-sized apps needing global state. Implements context splitting and combining with reducers for better performance.
-
Redux: Ideal for large-scale apps with complex state interactions. Utilizes middleware for side effects, selectors for derived state, and 'Redux Toolkit' for simplified logic.
Choose the approach based on your app's size and complexity. These methods can be combined as needed, starting simple and scaling up as the application grows.