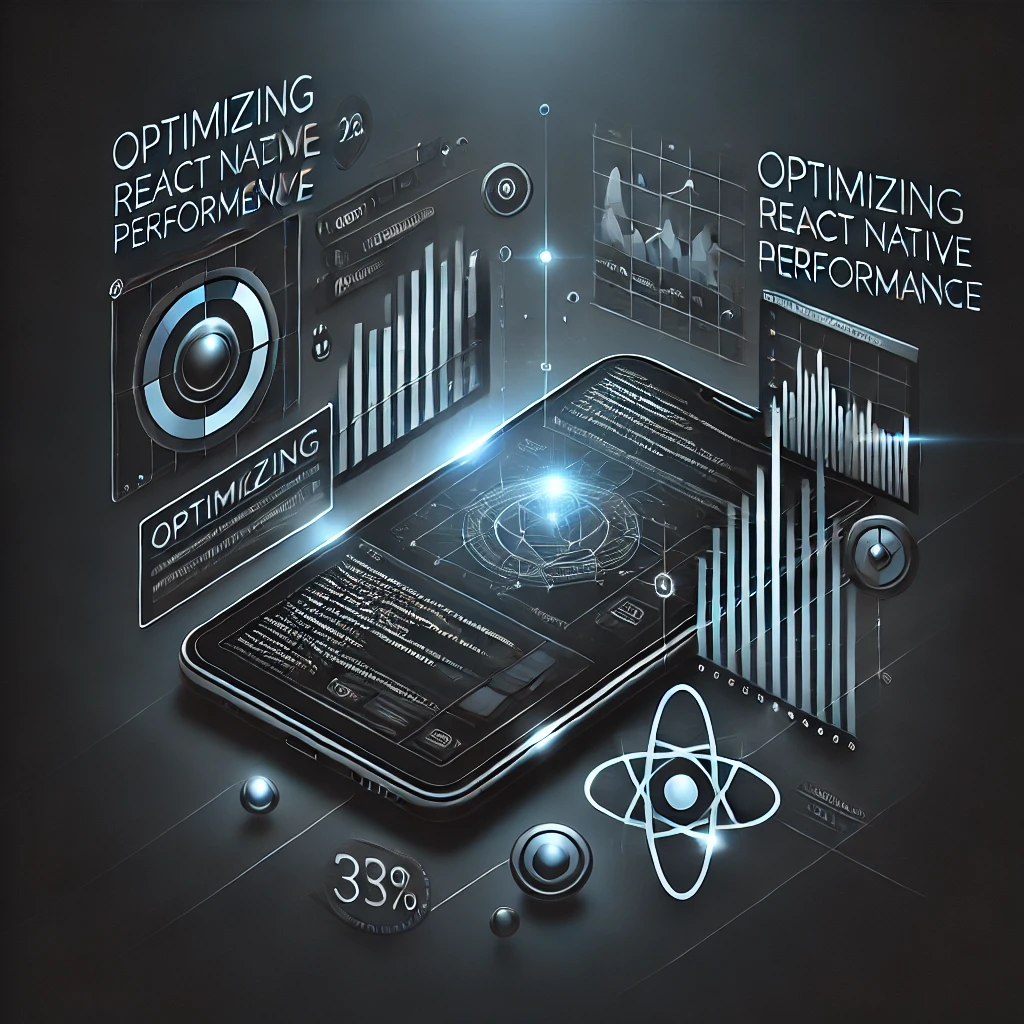
Optimizing React Native Performance
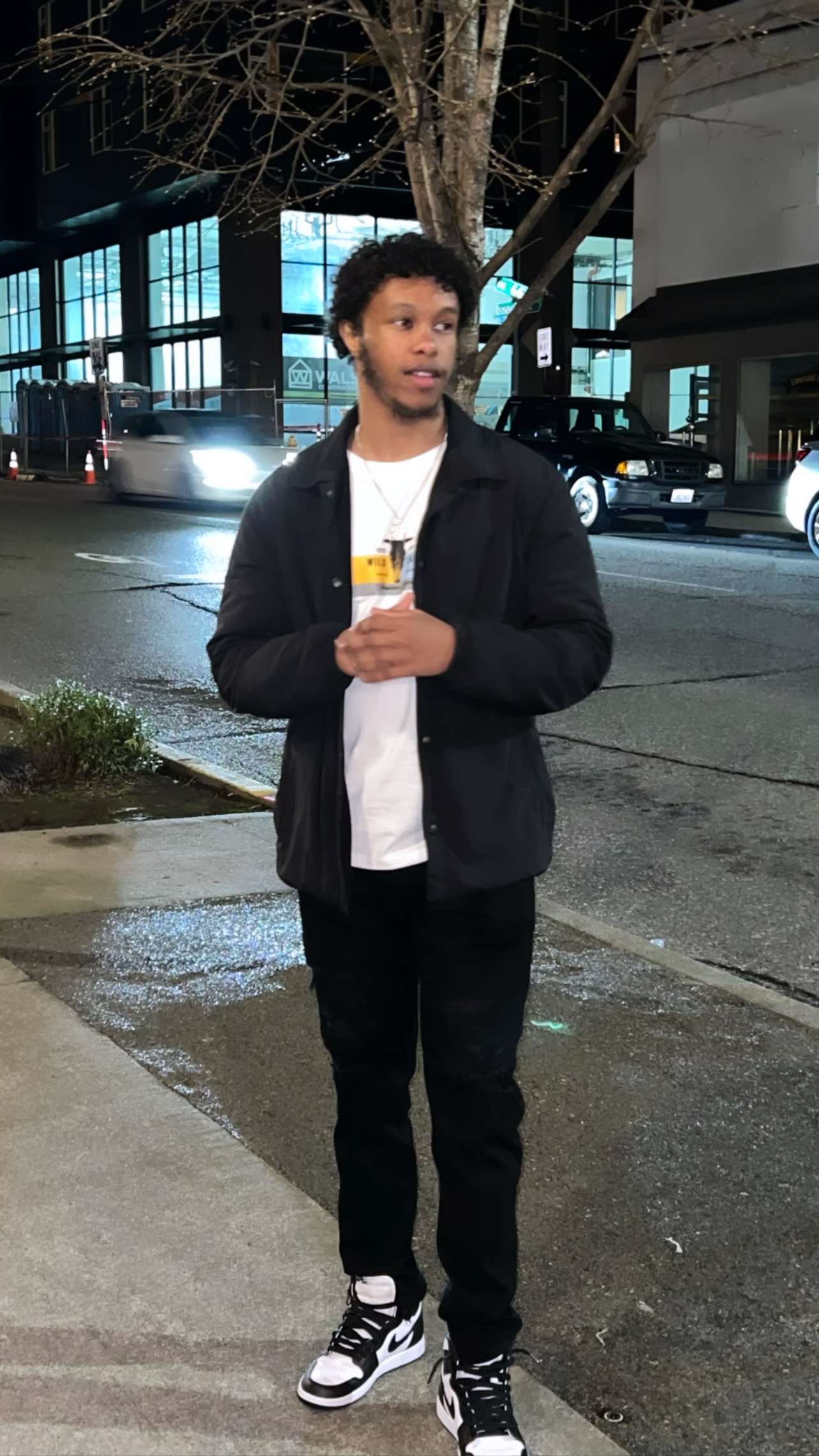
Najib Abdi, October 16, 2024
React Native offers a robust framework for developing cross-platform mobile applications efficiently. However, achieving optimal performance requires more than just leveraging its core features. Effective optimization is essential to ensure a seamless and responsive user experience. In this guide, we'll cover some essential tips and best practices to help you get the most out of your React Native APP.
1. Use useMemo
and useCallback
Wisely
One common performance issue in React Native apps is unnecessary re-rendering of components. This can lead to performance bottlenecks and a sluggish user experience. To address this, you can use the 'useMemo' and 'useCallback' hooks to memoize values and functions, thereby preventing redundant renders.
import React, { useMemo, useCallback } from 'react';
import { View, Text, Button } from 'react-native';
const MyComponent = ({ data }) => {
// Memoize the processed data to avoid recalculating on every render
const processedData = useMemo(() => processData(data), [data]);
// Memoize the button handler to prevent re-creation on every render
const handlePress = useCallback(() => {
console.log('Button pressed!');
}, []);
return (
<View>
<Text>{processedData}</Text>
<Button title="Press me" onPress={handlePress} />
</View>
);
};
useMemo
This hook helps avoid recalculating the 'processData' value unless the data prop changes. By memoizing the result of the 'processData' function, you reduce unnecessary computations and improve performance.useCallback
This hook ensures that the 'handlePress' function is not recreated on every render. Instead, it remains stable unless its dependencies change, which helps avoid unnecessary re-renders of components relying on it.
2. Optimize List Rendering with FlatList
Handling large datasets efficiently is crucial for maintaining performance in React Native applications. 'FlatList' is designed to handle large lists more efficiently than 'ScrollView' by rendering only the items currently visible on the screen. This approach reduces memory usage and improves scrolling performance.
import React from 'react';
import { FlatList, Text, View } from 'react-native';
const MyList = ({ data }) => (
<FlatList
data={data}
keyExtractor={(item)=> item.id}
renderItem={({ item })=> <Text>{item.title}</Text>}
/>
);
Tips for FlatList Optimization:
- Use keyExtractor: This function helps React Native efficiently manage list items by providing a stable and unique key for each item. This improves list rendering performance and avoids potential issues with item reordering or updating.
- Avoid inline functions: Define 'renderItem' and other functions outside of the FlatList component. Inline functions can lead to unnecessary re-renders as they are re-created on every render pass.
- Use getItemLayout: If your list items have a fixed height, implement 'getItemLayout' to optimize rendering. This function allows React Native to calculate item positions without rendering the entire list, improving performance.
3. Avoid Anonymous Functions in JSX
Passing anonymous functions directly in JSX can cause unnecessary re-renders and degrade performance. To optimize, define these functions outside of the JSX and use memoization hooks when appropriate.
import React, { useCallback } from 'react';
import { Button } from 'react-native';
// Define the function outside of JSX
const MyComponent = () => {
const handlePress = useCallback(() => {
console.log('Button pressed!');
}, []);
return (
<Button title="Press me" onPress={handlePress} />
);
};
By defining 'handlePress' outside the render method and using 'useCallback', you ensure that the function is stable and does not cause unnecessary re-renders, thus improving performance.
4. Optimize Image Rendering
Images can significantly impact performance in mobile applications. Optimize image rendering to avoid performance issues and ensure smooth user experiences.
- Use Image.getSize: This method allows you to fetch the dimensions of remote images before rendering them. Knowing the image size in advance helps avoid layout thrashing and ensures that images are displayed correctly.
- Use resizeMode: Set the 'resizeMode' property to 'contain' or 'cover' to control how images are scaled and displayed. This prevents unnecessary scaling and ensures that images fit well within their containers.
import { Image } from 'react-native';
<Image
source={{ uri: 'https://example.com/image.jpg' }}
style={{ width: 100, height: 100 }}
resizeMode="contain"
/>
5. Minimize the Use of Inline Styles
Inline styles can be problematic as they are re-created on every render, leading to performance inefficiencies. Instead, use 'StyleSheet' to define styles outside the render method for better performance.
import { StyleSheet, Text, View } from 'react-native';
const styles = StyleSheet.create({
container: {
padding: 10,
backgroundColor: 'white',
},
text: {
color: 'black',
},
});
const MyComponent = () => (
<View style={styles.container}>
<Text style={styles.text}>Hello, World!</Text>
</View>
);
By using 'StyleSheet.create', styles are only created once, which minimizes the overhead of style object creation and improves rendering performance.
6. Monitor Performance with react-native-performance
To keep track of and optimize your app's performance, the 'react-native-performance' library provides detailed insights into various aspects of performance, including component rendering times and network requests.
6.1 Install the Library
First, install the 'react-native-performance' library using npm or yarn. This library will provide the necessary tools for performance monitoring.
npm install react-native-performance
6.2 Initialize the Library
Initialize 'react-native-performance' in your app's entry point file. This step sets up the performance monitoring tool to start tracking metrics.
import { PerformanceMonitor } from'react-native-performance';
// Initialize the performance monitor
PerformanceMonitor.start();
6.3 Use Performance Monitoring Tools
Monitor how long components take to render by using the measure function. This helps identify performance bottlenecks related to specific components.
import React, { useEffect } from'react';
import { Text, View } from'react-native';
import { measure } from'react-native-performance';
constMyComponent = () => {
useEffect(() => {
const stop = measure('MyComponentRender');
return() => {
stop(); // Stop measuring when the component unmounts
};
}, []);
return (
<View><Text>Hello, World!</Text></View>
);
};
Summary
By implementing these best practices and utilizing performance monitoring tools like react-native-performance, you can significantly enhance the performance of your React Native apps. Regularly monitor and optimize your app to ensure a smooth and efficient user experience.